82.删除排序链表中的重复元素 II
【LetMeFly】82.删除排序链表中的重复元素 II:模拟
力扣题目链接:https://leetcode.cn/problems/remove-duplicates-from-sorted-list-ii/
给定一个已排序的链表的头 head
, 删除原始链表中所有重复数字的节点,只留下不同的数字 。返回 已排序的链表 。
示例 1:
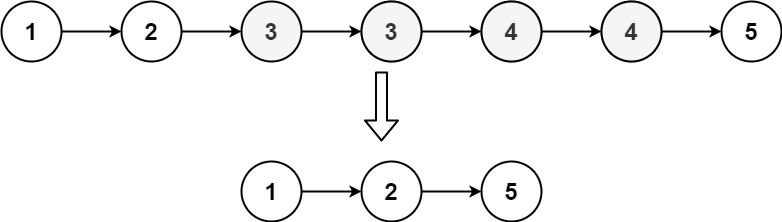
输入:head = [1,2,3,3,4,4,5] 输出:[1,2,5]
示例 2:
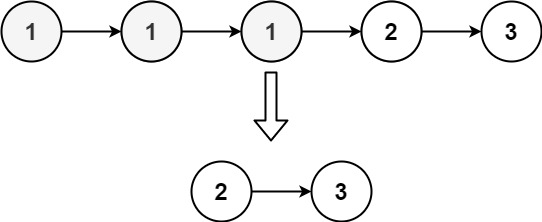
输入:head = [1,1,1,2,3] 输出:[2,3]
提示:
- 链表中节点数目在范围
[0, 300]
内 -100 <= Node.val <= 100
- 题目数据保证链表已经按升序 排列
方法一:模拟
相同的节点可能被全部删除(头节点可能也会被删),因此我们可以新建一个“空的头节点ans”,ans的next指向head。
使用两个节点lastNode和thisNode,lastNode指向上一个节点(防止当前遍历到的节点被删除),thisNode指向当前处理到的节点。当thisNode和thisNode.next都非空时:
- 如果
thisNode.val == thisNode.next.val
,新建一个nextNode节点指向thisNode.next.next(最终指向第一个和thisNode的值不同的节点)。当nextNode非空且nextNode.val == thisNode.val
时,nextNode不断后移。最后将lastNode.next赋值为nextNode,并将thisNode赋值为nextNode(删掉了中间具有相同元素的节点)。 - 否则,将lastNode和thisNode分别赋值为thisNode和thisNode.next(相当于指针后移)
最终返回“假头节点”ans的next即可。
- 时间复杂度$O(len(listnode))$
- 空间复杂度$O(1)$
AC代码
C++
1 |
|
Python
1 |
|
同步发文于CSDN,原创不易,转载经作者同意后请附上原文链接哦~
Tisfy:https://letmefly.blog.csdn.net/article/details/135612345
82.删除排序链表中的重复元素 II
https://blog.letmefly.xyz/2024/01/15/LeetCode 0082.删除排序链表中的重复元素II/